Battler Flipbooks Core MV + MZ
A downloadable RPG Maker plugin
This plugin provides infrastructure to temporarily replace a battler's sprite with a flipbook animation, loading these animations reliably, and for transitioning between the animations seamlessly.
This is a core-only plugin. To use it, additional JavaScript plugins are required.
Before deploying for Linux on Windows, you must update MV's NW.js runtime.
There should be enough documentation, including basic parameter structure definitions, to DIY a controller for your use-case if you're familiar with RPG Maker MV or MZ plugin development and JavaScript classes, but I hope you will still consider my premade add-in and layer plugins for this system. They are priced in a way that buying them should be immediately worth the time you save on the initial implementation of each provided feature.
This page details common functionality implemented by the Core, which is shared by most of my layers (here in recommended load order):
- Conditional Battler Flipbooks: TBA
- Battler Idle Fidget Flipbooks: TBA
- Battler Action Flipbooks: TBA
Additional features can be made available to all layers at once by add-ins like the following:
- Battler Flipbooks Delay Loaded: TBA
- Battler Flipbook Callback Events: TBA
- Meta Conditionals for Battler Flipbook Events¹: TBA
¹ Event conditionals are technically possible with just the core, so this will be about making them easier to write and providing composable meta events. This won't be included with the Events Core due to the feature's relative complexity.
(I am splitting these up and staggering the release to make this system more affordable and easier for me to manage. The whole project is multiple hundred hours of work, so basic access would be too expensive for my taste if priced reasonably in a single package.)
General Hints
- You can double-click the divider between the Name and Status columns to see the full plugin names quickly.
- Start simple! Even a single-cel pose can look great if displayed for the right amount of time.
- For short or fast animations, use smaller differences or less detailed cels.
- For larger pose changes and more complex animations, make sure the frames are visible long enough that someone less familiar with them can parse most of them!
- If the animation appears very often, you can use a short cel delay with more detail to keep it interesting, but still make it easy to get the gist.
- You can duplicate cels with Ctrl+C, Ctrl+V to display them longer than others in the same list. This does *not* hurt performance!
- Shorthand expansions are not customisable in the plugin parameters, but are usually easy to edit in the respective plugin source code.
- Search for
expandShorthand
. - You can use https://regex101.com/ to debug regex expressions if you select ECMAScript mode there.
- Search for
Flipbooks Core Cheat Sheet
Timing / Hooks
Largely determined by dependent plugins, but resources are released automatically on Scene_Battle.prototype.terminate
(i.e. whenever a battle ends).
JS Callback Arguments
Purpose | Identifier | Type |
---|---|---|
Who or what may be animated? | battler | Game_Battler |
Which are they? | index | number |
Shorthands
Category | Subcategory | Identifier | Type |
---|---|---|---|
Game State¹ | Switches | Sw…² | boolean |
Variables | Var…² | number | |
battler | State | St…², isDeathStateAffected, isDualWield, isAutoBattle, isGuard, isSubstitute, isPreserveTp, isHidden, isAppeared, isDead, isAlive, isDying, isRestricted, isConfused, isActor, isEnemy | boolean |
Options | canInput, canMove, canAttack, canGuard | boolean | |
Buffs & Debuffs¹ | mhpBuff, mmpBuff, atkBuff, defBuff, matBuff, mdfBuff, agiBuff, lukBuff, anyBuff | number | |
Buff State¹ | mhp…, mmp…, atk…, def…, mat…, mdf…, agi…, luk…, any… …Is… …BuffAffected, …DebuffAffected, …BuffOrDebuffAffected, …MaxBuffAffected, …MaxDebuffAffected, …BuffExpired | boolean | |
Properties | hp, mp, tp, mhp, mmp, atk, def, mat, mdf, agi, luk, hit, eva, cri, cev, mev, mrf, cnt, hrg, mrg, trg, tgr, grd, rec, pha, mcr, tcr, pdr, mdr, fdr, exr | number | |
Phase¹ | isSelected, isUndecided, isInputting, isWaiting, isActing, isChanting, isGuardWaiting | boolean |
¹ Translated to method calls.
² where … is an integer.
Animation Callback Arguments
These are available in the Home override... coordinate expressions, in addition to the general JS Arguments and Shorthands.
Category | Type | Identifier | Explanation |
---|---|---|---|
animated sprite | Sprite_Battler | sprite | |
current animation | FlipbookAnimation | animation | |
timing info³ | number | frame | Logical animation frame index, negative during the intro. |
timelineFrame | Same as frame , but each repeat occupies the same range starting at 0, followed directly by the outro. | ||
tSections | Fractional number from -1.0 to 2.0, where the intro spans -1.0 to just before 0.0, each repeat spans 0.0 to just before 1.0 and the outro spans 1.0 to just before 2.0. | ||
tFull | A fractional number from 0.0 to just before 1.0, tracking the progress through the animation's full undisturbed runtime. |
³ Timing info values may fall outside the given ranges when observed under different circumstances (usually through additional plugins)!
Timing info values may skip or not reach all values when an animation is interrupted. frame
and tFull
are likely to skip part of their unrolled repeating section after an animation is scheduled to end early.
frame
and timelineFrame
may well be non-integer numbers (except during BattlerFlipbookState..advance
, as noted by plugins where this matters)!
Filter Infrastructure
The following filters will usually be available across layer plugins to determine which flipbook is applied to a given battler in the respective situation:
- By actor or enemy, directly or by custom note tag.
- By tags of the preceding flipbook (globally across layers).
- Also exclusions.
- By equipped weapon(s), also by type(s), optionally also for enemies.
- By equipped armour(s), also by type(s), optionally also for enemies.
- By States the battler is affected by.
- Also exclusions.
- By Switches.
- Also exclusions.
- By free-form shorthand and/or plain JavaScript condition.
Effect Infrastructure
The following optional side-effects are available across most of my layer plugins, configurable per flipbook:
- Flipbook tags, a powerful system to determine which animations can follow and interrupt each other.
- This gives you nearly the power of animation state machines in more generic game engines, in terms of transitioning animations smoothly into each other.
- Sprite home position override according to a formula, either additive or absolute.
- Cycle interruptions of lower-priority layers, allowing you to e.g. jump into a flinch without delay but transition out of it seamlessly into an idle animation.
- Blocked sprite effects, like 'whiten', 'blink', 'collapse', 'bossCollapse' and 'instantCollapse'. This stops the engine from interfering with your animated enemies.
- Blocked sprite motions, like 'guard', 'spell', 'skill', 'item', 'thrust', 'swing', 'missile', 'damage', 'evade', 'victory' and 'escape'. This turns off specific side-view actor spritesheet animations when needed.
Animation Infrastructure
Each animation is normally made up of three parts, each (or all!) of which may be empty and can have distinct frame delays:
- an intro
- a repeated middle section (with configurable repetition limit)
- an outro
Battler Flipbooks Core provides a consistent animation timeline interface for use by add-ins and layers alike and can dynamically shorten an animation by omitting further repetitions, which makes it easier to create controller plugins that maintain the flow of battle.
Additionally, the common playback speed of animations can be varied dynamically alongside RPG Maker's built-in fast-forward feature or by hooking this plugin's API's static speed
getter, even at fractional rates, without affecting individual animation playback logic.
Animations' resources are reserved and loaded in the background during each battle, and playback can configurably wait until the respective animation is ready, so playback is reliable without increasing initial battle load times.
Caution
This is not a video sprite streaming plugin. This means that using a large number of distinct animation frames can require a large amount of memory. (Reused cels are reused even across distinct layers, however.)
Refer to each controller plugin's documentation for more information on memory use and optimisation options.
Load Order
This plugin (Battler Flipbooks Core) must be loaded after any plugins that reimplement the battle system from scratch, like YEP_BattleEngineCore or (most likely) VisuMZ_1_BattleCore.
Layers should be loaded after status pose plugins like YEP_X_WeakEnemyPoses to have higher priority than them.
Recommended load order for my Battler Flipbooks plugins:
Filename | Description |
---|---|
TS_Battler_Flipbooks_Core | … |
… | (B.Fl. Add-in) … |
TS_Battler_Flipbook_Events_Core | (B.Fl. Add-in) … |
… | (B.Fl. Events Add-in) … |
… | (B.Fl. Layer) … |
All add-ins should be loaded before all layers (that they should interact with), as they may be used during layer instantiation.
The order of add-ins usually doesn't matter.
Layers should be ordered from least to most situational - for example, you should load "Entrance Flipbooks" first and then "Reaction Flipbooks" below it, so that the latter has higher priority and can correctly interrupt a battler's default idle loop (if Entrance Flipbooks is used that way).
Plugin Parameters
Default speed:
Multiplier for how quickly to play flipbook animations by default during battle.
There can be a little (sub-frame) drift when animations end if using speeds that aren't a whole-number fraction of 1, but this shouldn't be noticeable.
Fast-forward speed:
Multiplier for how quickly to play animations, applied when fast-forwarding during battle. (By default when holding "Shift" or "Enter".)
There can be a little (sub-frame) drift when animations end if using speeds that aren't a whole-number fraction of 1, but this shouldn't be noticeable.
Limit frame skip:
Limits performance-related frame skipping done by the RPG Maker renderer during battle.
This does not affect the game or animation logic, but may be helpful to avoid visually missing cels at high animation frame rates.
Iff enabled, use the "Limit:" parameter to control how many game frames may still be skipped for each frame that is shown on screen. This should be lower than the lowest animation frame delay you want to ensure is visible.
Plugin Commands
This plugin does not expose any plugin commands.
JavaScript API
(You can skip this part unless you'd like to make custom layers or add-ins.)
This plugin unconditionally sets the global variable TS_Battler_Flipbooks_Core
when first loaded, through which all API members are available.
For detailed JS API endpoint documentation, please refer to the type annotations and JSDoc comments inside the .d.ts file for this plugin.
The plugin source is unobfuscated and not precompiled, so it should be very readable too, if necessary.
For Layers
You can find an example layer plugin under CC0 here on GitHub, which should make it easier to get started.
- Layers should create derived classes extending each of
HomeOverride
,FlipbookAnimation
,Flipbook
,FlipbooksRule
andFlipbooksRuntime
.BattlerFlipbookState
should usually not be extended this way.- Override the static methods at the top of each class to introduce the derived classes to each other, where applicable!
FlipbooksRule
can be initialised from parsed plugin parameters - there are matching RPG Maker plugin parameter structures and a decodeParameters helper function to get you started in this regard.- I recommend adding parameters that control conditions to the rule and parameters that control effects to the flipbook structure, to keep everything consistent between different layer plugins.
Once the rules are hydrated into live objects, the layer can instantiate its runtime class using these rules and any additional parameters. It should then expose an API containing its parameters, class constructors, hooks (for example as ...core.makeHook(() => api, …),
, if this plugin here is available as core
and the constructed hook holder will be api
) and any other methods necessary to interact with and extend that plugin.
Note: When overriding methods, make sure to still call the super.
method even if it is empty! This ensures that add-ins will function correctly with your layer plugin.
For Add-ins
Add-ins will generally hook the core's API methods to change their behaviour or add features that should be available in all layer plugins.
Hint: The .prototype.initialize
methods are good places to parse note tags only once. Make sure that your custom code executes *after* the original implementation, then examine this.note
. Like with the other validated properties, you can assume that this field will have been initialised to its correct type at this point.
Hint: If needed, you can also use .prototype.initialize
to late-hook other prototype methods to manipulate their derived implementations. However, if you do so, make sure to apply the hook only once for each this.constructor
and to use a recursion flag to avoid running your hook twice! Additionally, please do expose a method that other plugins can hook to subscribe to your hook holder objects if you do go ahead with this approach.
Compatibility Notes
This plugin was tested on RPG Maker MV 1.6.1 and RPG Maker MZ 1.6.1, uses only the public RPG Maker API as far as possible, and does not use any platform-specific APIs.
This plugin should be compatible with any deployment target available for RPG Maker MV and MZ, including web and most custom ones.
This plugin is compatible with YEP_BattleEngineCore (v1.51), as long as it is loaded after that battle engine.
Compatibility with VisuMZ_1_BattleCore (Version 1.73) is *likely*, but hard to judge conclusively due to its obfuscated source code. (Not great.)
If you notice issues or glitches in combination with other plugins, please tell me about them, and I'll check if a compatibility tweak is feasible.
This plugin is compatible with YEP_ItemCore (independent items).
Copy of License Grant
(as included in the plugin file, aside from line wrapping)
A license for this plugin can be acquired at https://tamschi.itch.io/battler-flipbooks-core .
Once you have acquired it, you may redistribute and sublicense this plugin file as part of games you create. You may not sublicense it separately or as part of an asset- or resource-collection.
You may modify this plugin when including it with your games, as long as the attribution above and this license grant stay intact. If you do so, you must add comments to indicate which changes you made from the original.
Additionally, you may redistribute this file alongside its matching .d.ts file, both only unchanged and free of charge under this same license, as long as you at least equally present the above link as the original download location as alternative to your copy of this file and make that link freely available without any additional login or membership requirement compared to viewing any advertisement of your copy of this file.
Additionally, you may copy and relicense the plugin parameter structure definitions below (That is: the block comments beginning with `/*~struct~`), as long as you, nearby in your plugin source code, mention their origin and point out your modifications.
Download
Click download now to get access to the following files:
Development log
- Ver. 1.2.4: Independent items compatibilityMay 19, 2024
- Layer example pluginMar 05, 2024
- Ver. 1.1.4Jan 25, 2024
- Ver. 1.1.3Jul 21, 2023
- Ver. 1.0.2Jun 27, 2023
- Ver. 1.0.1Jun 06, 2023
- Small page update (and upcoming patch)May 26, 2023
Comments
Log in with itch.io to leave a comment.
Hello (and sorry for not replying earlier. I’m currently away from home, so I can’t check back quite as often)!
I’ll try explain it in a way that translates well. My answer also depends on whether you are able to write JavaScript plugins.
This “Core” here takes care of loading, running and displaying animations, but it doesn’t know when to start or shorten animations. At least one “Layer” is needed, which triggers animations based on the flow of battle.
(I broke this into multiple parts to make it more affordable, as for many games, just one or two Layers are needed.)
If you’d like to use a premade Layer (without writing JavaScript), I recommend Occasional Battler Flipbooks in most cases. This Layer allows you to have attack, damage, miss, evade and K.O. animations, among others. It can’t distinguish different attacks or damage types, however. It also can’t use contextual parameters like the damage amount. Please check the video on the page to see if this fits your use-case.
To also (or only) add frame-based entrance and idle animations, I recommend Battler Entrance Flipbooks. This Layer starts a single animation along with the battle and can also delay the start of battle until after the entrance animations.
Layers stack, so if you use both, then “occasional” animations can interrupt the idle animation, which then resumes once the “occasional” animation is over. You can see an example of this in the video for Battler Flipbook Sound Effect Events, which is an “Add-In” that adds sound effects to any Layer. You can keep using the default sound effect system too, of course.
I can’t offer translations for the help text, since I can’t afford a professional translation. Machine translation usually doesn’t work well with this kind of technical text.
However, if it would be helpful, I could try to machine-translate the parameters and their descriptions starting around the end of next week. I would be very thankful if you could point out errors, in that case.
If you know how to write your own plugins in JavaScript, you could also create your own Layer plugin instead of using one of mine.
Layers usually have one parameter where rules are entered, like this:
(The parameter definition of
FlipbooksRule
and nestedstruct
s is included in Battler Flipbooks Core’s source code and must be copied into each Layer.Most Layers change it somewhat since they have additional conditions or effects.)
Then, in JavaScript, you can parse the parameters and instantiate the runtime like this:
Then, you can apply the rules at any time (normally in a hook) by calling
runtime.apply(battler, {})
, which will start applicable animations.This here is (roughly) what runs the animations in Battler Entrance Flipbooks:
core.makeHook
is a helper function I use to let other plugins easily change my hooks. You can hook an engine function plainly instead like this:(This is quite minimal, but enough to run idle animations. I can make a starter project for layers around the end of next week and upload it here under a permissive license.)
I was able to publish the example plugin earlier than expected: https://tamschi.itch.io/battler-flipbooks-core/devlog/692886/layer-example-plugin
This is a small script that only shows damage animations, but it can be modified to play animations for other events.
(If this already solves your problem, I would greatly appreciate a small support payment, as creating polished plugins like Battler Flipbooks Core takes a lot of work. Only if you can spare it, of course.)
Sorry for checking late!!(ㅠ.ㅠ) I was busy doing the graduation game jam. Thank you so much for your kind reply. I want to pay the application fee, but I don't have an account. I will definitely be happy once the account is created. I will apply it as instructed. Thank you again!!
Please don’t stress out over it, but thank you, any amount really helps.
Battler Flipbooks Core can be used for free, so please first make sure it works for what you’d like to use it for. (There is an option to not pay anything after you use the “Download now” button on this page.)
If you’re satisfied, you can use the button again and enter the amount that feels right to you.
If you decide on an amount that would be enough to purchase one of my paid plugins, I recommend making that purchase instead, since that way you’ll get another useful plugin 🙂
(It really is fine to do this, since my compensation doesn’t depend at all on which one you choose if you enter the same amount.)
And just to make sure there’s no misunderstanding: You don’t need a GitHub account to use the template. First click on
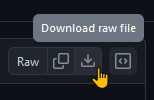
Battler_Flipbooks_LAYER.js
, then on this button above the file content to download it to your computer:You can then save it in your plugins/ folder and edit it with any plaintext editor if you need to make changes.
Screenshots and demo is possible? Is very confusing to imagine its potential. Also, something for animations in map? For action or SRPG battle engines (animation in normal map, outside battle map, no battler)? Thanks
It’s tricky because this is the kind of generic/subdued system that looks and behaves entirely differently depending on how it’s used. I have a very unpolished recording from about a month ago here with some features and fixes still missing at that point, as I had to make rough versions of some extensions to figure out and test the core, but this may not reflect well how someone else would use it in practice.
I’ll still try to make a better showcase once I have more of my code polished and published… but it will still feature those programmer art cubes or similar because I really can’t draw well enough for more than that.
Regarding map animations: The main reason this plugin is able to work efficiently and seamlessly at the same time is that it can predict which animations may be used based on the battlers that are present. Keeping all animations for all battlers in your game always in memory would likely cause issues due to memory use, especially on mobile/web, but they need to be in memory to play instantly when needed.
Map events also don’t (easily) have the meta data I use for flipbook rule conditions, and I can’t set up a nice object selector for them in the plugin parameters afaik, so animating them directly this way isn’t that feasible from a game content creation point of view.
The good news is that at least some map battle engines do in fact use battlers and
Window_BattleLog
internally, which makes them somewhat nearly compatible with this system already!What’s needed to make it work is another plugin loaded after Battler Flipbooks Core that bridges it to the map battle system loaded earlier, and adjusts
Sprite_Character
to show bitmaps from a hiddenSprite_Battler
-like instance animated this way during battles.I’m close to certain that this is possible without changes to Battler Flipbooks Core, since all engine hooks are published as before/after properties and can be adjusted and called as needed without unintended side-effects due to that.
However, I currently don’t have plans to implement this myself. I am available for questions and code review towards this purpose though (and related to Battler Flipbooks in general, really).
Saw video and I think is very interesting, I can imagine many uses now, thank you. I will follow future updates with a lot of interest.
Thank you for the follow :)
Note that there will be a bit of overlap between the layers I make, since there’s a trade-off between how different the battle events that can trigger animations in a layer can be and how well the rules can react to specific context of an event.
For example, Battler Reaction Flipbooks will let you filter conveniently by how much damage an action did as percentage of the target’s max HP or its elemental effectivity, among many other action-related conditions, and can be used to make that damage-scaled fake knockback you saw in the video.
However, if you need only a simple general damage reaction per battler, then Occasional Battler Flipbooks will be enough, since it can be used to play an animation whenever the
Game_Battler.prototype.performDamage
engine function is called.That one will come with a handy drop-down with suggestions for about 160 battler-related engine functions it can dynamically hook into, but without the additional filter conditions available in more specific layers.